Suspense for Data Fetching Experimental React
Contents
Most people on the web are used to page transitions taking some amount of time to load. You also tend to be re-rendering the entire page at once so your users are unlikely to be interacting with other elements on the page at the same time. React.lazy takes a function that must call a dynamic import(). This must return a Promise which resolves to a module with a default export containing a React component.
Feedback to data revalidationNow, we can see the data being reused instead of rendering the componentSkeletonLoading. But, we still provide feedback while the data is revalidating, two times. One by displaying the spinner next to the most important text and other by disabling the button that performs the revalidating action. This time the hookuseSWR will not throw an exception that Suspense handles. This hook will reuse the data (now the name makes sense, right?) and expose a variable that enables us to perform this revalidation.
createFetchStore
SWR offers plentiful options for revalidating the data automatically. Expect these to be work for suspend-react once they come out. Since suspend operates on a global cache , you might be wondering if keys could bleed, and yes they would. To establish cache-safety, create unique or semi-unique appendixes.
The difficulty we’re experiencing is “synchronizing” several processes in time that affect each other. Note how we eliminated the if (…) “is loading” checks from our components. This doesn’t only remove boilerplate code, but it also simplifies making quick design changes. For example, if we wanted profile details and posts to always “pop in” together, we could delete the boundary between them. Or we could make them independent from each other by giving each its own boundary.
- It will not delay a render if it’s the result of an urgent update.
- This Promise argument is usually going to be a network request to retrieve some data from an API, but it could technically be any Promise object.
- We’re going to fetch user data and a profile picture from JSONPlaceholder mock endpoint.
- They have exposed 2 React lifecycles, static getDerivedStateFromError() and componentDidCatch().
- In this section, we’ve seen how React suspense can be used in multiple data fetching components.
- If you’re using Create React App, Next.js, Gatsby, or a similar tool, you will have a Webpack setup out of the box to bundle your app.
When the resource finally loads, React will try to render the component again. React suspense is a ReactJS technique that enables data fetching libraries to inform React when asynchronous data for a component is still being fetched. It suspends the component from rendering until the required data is obtained and provides a fallback UI during the fetch duration. It is important to note that Suspense is not a data fetching library like react-async, nor is it a way to manage state like Redux.
Building Great User Experiences with Concurrent Mode and Suspense describes why this matters and how to implement this pattern in practice. Practically speaking, is a first-party React component used to wrap other components that might make asynchronous requests. Any time a child component performs some action resulting in a loading state, such as a network request, a wrapping component can toggle its rendering to show a loading UI, like a . In the example below, the Albums component suspends while fetching the list of albums. Until it’s ready to render, React switches the closest Suspense boundary above to show the fallback—your Loading component.
We could remove the Promise.all() call, and wait for both Promises separately. However, this approach gets progressively more difficult as the complexity 10 Top Cloud Security Companies of our data and component tree grows. It’s hard to write reliable components when arbitrary parts of the data tree may be missing or stale.
This allows you to solve the problems we encountered with the other approaches in a trivial manner. It lets us begin rendering our component immediately after triggering the network request. In this case, we’ve Bottom Up Mergesort GitHub moved the fetching logic outside of the App component so that the network request begins before the component is even mounted. Another change we made is that no longer triggers its own async requests.
FetchData.js, on the other hand, contains a function that fetches data from a server using the Fetch API, and returns a promise wrapped with the wrapPromise function. Next, we create a new function called read, and inside this function, we have a switch statement that checks the value of the status variable. If the status of the promise is “pending,” we throw the suspender variable we just defined. And, finally, if it is anything other than the two (i.e., “success”), we return the response variable.
Todos.jsx
Any time a child component throws an error, such as a failed network request, a wrapping Error Boundary component can toggle its rendering to show a custom error UI. Although it’s technically doable, Suspense is not currently intended as a way to start fetching data when a component renders. Rather, it lets components express that they’re “waiting” for data that is already being fetched.
Unfortunately, the latest version of React has one of those. Declarative programming, on the other hand, is typically easier to write. It means instead of having to figure out what order everything should be done in, we state our intention of what we want, and we let the application figure it out for us.
As a data fetching library author, you can enforce this by making it impossible to get a resource object without also starting a fetch. Every demo on this page using our “fake API” enforces this. In this section, we’ll create an error boundary component for handling errors in our components. The error boundary component will handle both rendering errors and errors from suspense data fetching. Next, let’s explore some common data fetching approaches, their limitations, and how Suspense improves the developer and user experience.
An example of the difference between Concurrent React, rendering a page, and the React rendering engine prior is what happens when a web page updates a list based on some interruption . We’ve now covered the basics of Suspense for Data Fetching! Importantly, we now better understand why Suspense works this way, and how it fits into the data fetching space. In the long term, we intend Suspense to become the primary way to read asynchronous data from components — no matter where that data is coming from. This page describes experimental features that are not yet available in a stable release.
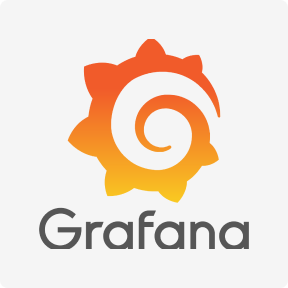
React skips over it, and tries rendering other components in the tree. Replacing visible UI with a fallback creates a jarring user experience. This can happen when an update causes a component to suspend, and the nearest Suspense boundary is already showing content to the user.
For instance, when adding a search feature, you might want to show the user what they are entering but wait to start the search until the user finishes typing. One of these enhancements involves React’s “Suspense” functionality. The “Suspense” feature has been part of the library since React 16. With the recent release of React 18, the capability has been enhanced even better. Avoid, as much as you can, to force the user to refresh the page. As a software engineer, you must provide the correct feedback at the right time by giving alternatives with scape actions in a stressful situation.
What Suspense Lets You Do
I previously wrote an article about how to code splitting a React app at the router level. React Suspense is a great way to improve the load time of your UI. In this post, learn about Suspense and why you should implement it in your next project.
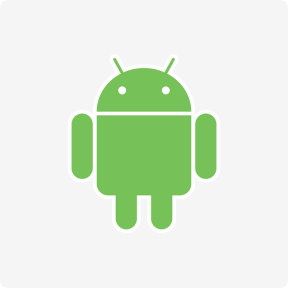
But you can use a library that’s integrated with Suspense . Suspense does not detect when data is fetched inside an Effect or event handler. Download the videos and assets to refer and learn offline without interuption. Refine is a React-based framework for the rapid development of web applications. It eliminates the repetitive tasks demanded by CRUD operations and provides industry standard solutions. Using components that handle concurrency using useEffect() inside Suspense.
Ready to skill upyour entire team?
React 18, also known as the Concurrent React, released earlier this year and brought with it important changes. The most impactful one is the new concurrent rendering engine, which is what the new Concurrent Rendering feature “Suspense” is based on. If your React apps work with any asynchronous data sources , using Suspense will not only make your apps easier to program, but those apps will also perform much better for your users. None of the data is fetched yet, so this component “suspends”.
Replies to “Experimental React: Using Suspense for data fetching”
The React.lazy function lets you render a dynamic import as a regular component. When Webpack comes across this syntax, it automatically starts code-splitting your app. If you’re using Create React App, this is already configured for you and you can start using it immediately. To avoid winding up with a large bundle, it’s good LRU Cache in Python using OrderedDict to get ahead of the problem and start “splitting” your bundle. Code-Splitting is a feature supported by bundlers like Webpack, Rollup and Browserify (via factor-bundle) which can create multiple bundles that can be dynamically loaded at runtime. At the moment React.Suspense works only with dynamic import() aka lazy loading.
We’re going to fetch user data and a profile picture from JSONPlaceholder mock endpoint. Both the ErrorBoundary and Suspense components have a fallback option. If it is, however, it will return the resolved data from the Promise, which, in this case, would be an array of todo items. We then go ahead to map over this array and render each to-do item.